
Introduction
In this professional guide, we’ll walk you through the steps needed to build a Python-based arbitrage bot. This bot will help identify arbitraging opportunities by tracking crypto prices and other useful metrics across exchanges. Please keep in mind that this guide focuses on identifying arbitrage opportunities and does not delve into the complexities of executing trades on exchanges. We’ll utilize the CoinGecko API to gather necessary data. The API is free to access but does require keyed authentication.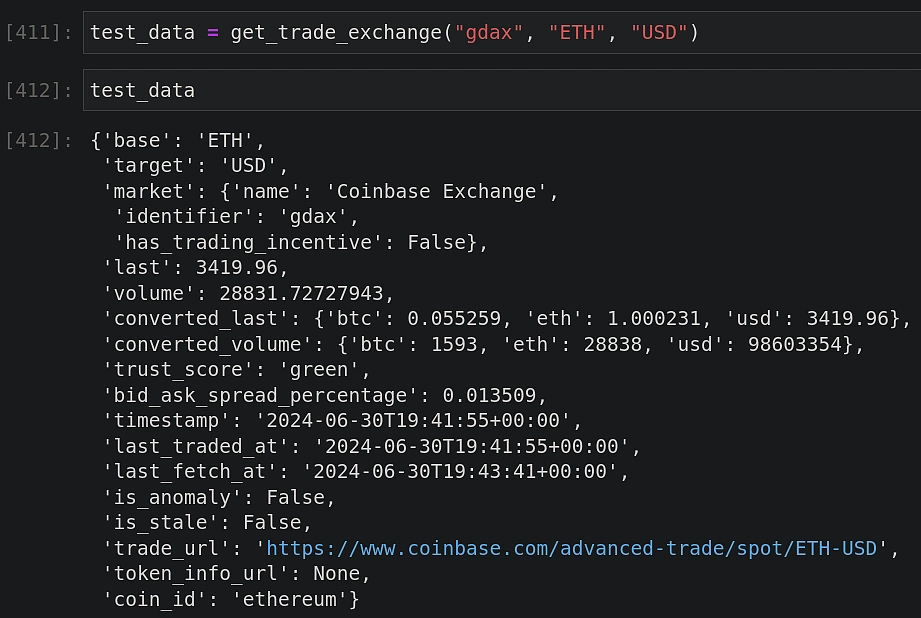
Prerequisites
To get started, there are several prerequisites you’ll need to satisfy:Software and Libraries
You’ll need Python 3. Additionally, the following Python packages must be installed:jupyterlab
pandas
numpy
pytz
Setting Up Jupyter Notebook
To start coding in a new notebook, execute the following command: jupyter lab This will launch a Jupyter notebook interface within a new tab of your default web browser.Setting Up the Project Environment and API Access
In your Jupyter notebook, start by loading the necessary Python libraries and setting up your environment. Here is a sample code snippet to get you started: import pandas as pd import numpy as np import requests from datetime import datetime from pytz import timezone import time from IPython.display import clear_output pd.set_option(‘display.max_rows’, 100) pd.set_option(‘display.max_columns’, 20) pd.set_option(‘display.width’, 1000)API Key Access
To access the CoinGecko API, you first need to generate an API key. Save this key locally and read it in your notebook: with open(‘coingecko_api_key.txt’, ‘r’) as file: api_key = file.read().strip() Define a helper function for making API requests: def get_response(endpoint, params=None): headers = { ‘accept’: ‘application/json’, ‘x-cg-pro-api-key’: api_key } response = requests.get(endpoint, headers=headers, params=params) if response.status_code == 200: return response.json() else: print(f”Error: “) return None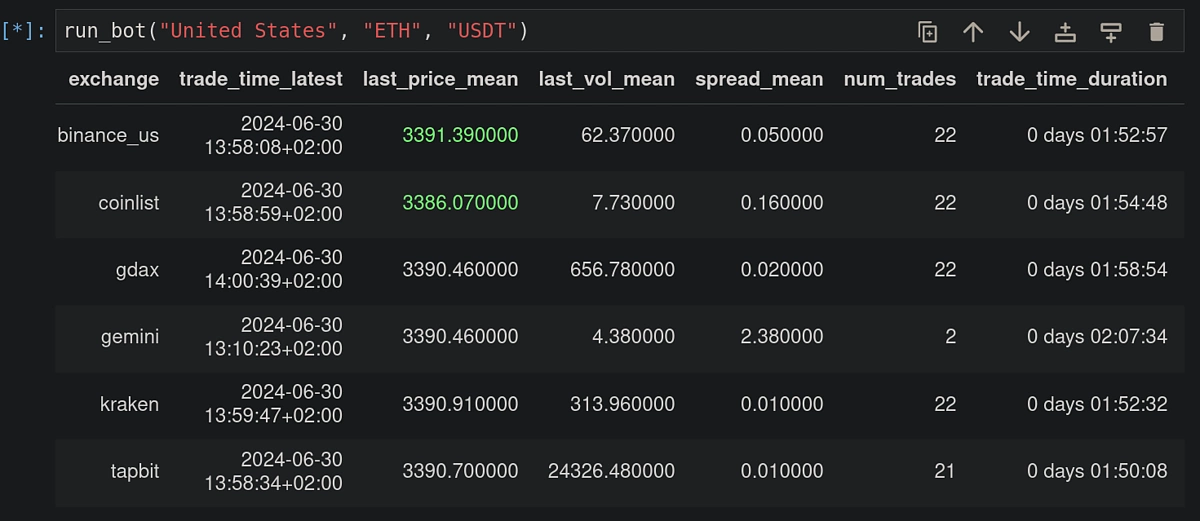
Retrieving Data from CoinGecko API
Listing All Crypto Exchanges
To get the list of all crypto exchanges, use the/exchanges
endpoint: endpoint = “https://api.coingecko.com/api/v3/exchanges” params = {‘per_page’: 250, ‘page’: 1} data = get_response(endpoint, params=params) exchanges_df = pd.DataFrame(data)Filtering and Sorting Exchanges
You can sort exchanges by trading volume or filter them based on their country of operation. Here’s how to filter US-based exchanges: us_exchanges = exchanges_df[exchanges_df[‘country’] == ‘United States’] us_exchanges = us_exchanges.sort_values(by=’trade_volume_24h_btc’, ascending=False) This will give you a DataFrame with exchanges in the US, sorted by their trading volume in BTC over the last 24 hours.Fetching Exchange Tickers
For each exchange, you can fetch trading pairs (tickers) using the/exchanges//tickers
endpoint: def get_ticker_data(exchange_id): endpoint = f”https://api.coingecko.com/api/v3/exchanges//tickers” data = get_response(endpoint) return data.get(‘tickers’, []) By combining these steps, you can gather data on a specific trading pair across multiple exchanges. For example, to get the price for the ETH-USD pair on Coinbase: tickers = get_ticker_data(‘gdax’) # id for Coinbase eth_usd_ticker = next((ticker for ticker in tickers if ticker[‘base’] == ‘ETH’ and ticker[‘target’] == ‘USD’), {}) print(eth_usd_ticker)Time Conversion
Data from APIs often comes in UTC. Use thepytz
library to convert timestamps to your local timezone: utc_time = datetime.strptime(eth_usd_ticker[‘timestamp’], ‘%Y-%m-%dT%H:%M:%S+00:00’) local_time = utc_time.replace(tzinfo=timezone(‘UTC’)).astimezone(timezone(‘Europe/Amsterdam’)) print(local_time)Monitoring Multiple Exchanges
Gathering Ticker Data
Extend the logic to gather ticker data across multiple exchanges. For enhanced analysis, you can filter exchanges by country and monitor specific trading pairs. selected_exchanges = us_exchanges[‘id’].tolist() ticker_data = [] for exchange_id in selected_exchanges: tickers = get_ticker_data(exchange_id) eth_usd_ticker = next((t for t in tickers if t[‘base’] == ‘ETH’ and t[‘target’] == ‘USD’), {}) if eth_usd_ticker: eth_usd_ticker[‘exchange’] = exchange_id ticker_data.append(eth_usd_ticker) else: print(f”Ticker not found on “) tickers_df = pd.DataFrame(ticker_data)Calculating the Spread
Calculate the bid-ask spread and other relevant metrics: tickers_df[‘spread’] = (tickers_df[‘ask’] – tickers_df[‘bid’]) / tickers_df[‘ask’] * 100 tickers_df = tickers_df.sort_values(by=’spread’)Conversion of Volume Data
Convert volume data from BTC to another currency using the/exchange_rates
endpoint: btc_to_usd_rate = get_response(“https://api.coingecko.com/api/v3/exchange_rates”).get(‘rates’).get(‘usd’).get(‘value’) tickers_df[‘converted_volume’] = tickers_df[‘trade_volume_24h_btc’] * btc_to_usd_rateHistorical Exchange Volume
Access historical volume data to understand trading trends and liquidity: def get_historical_volume(exchange_id, days=30): endpoint = f”https://api.coingecko.com/api/v3/exchanges//volume_chart” params = {‘days’: days} data = get_response(endpoint, params=params) return pd.DataFrame(data, columns=[“timestamp”, “volume”]) kraken_volume_df = get_historical_volume(“kraken”) Calculate a moving average of volume: kraken_volume_df[‘volume_ma’] = kraken_volume_df[‘volume’].rolling(window=7).mean()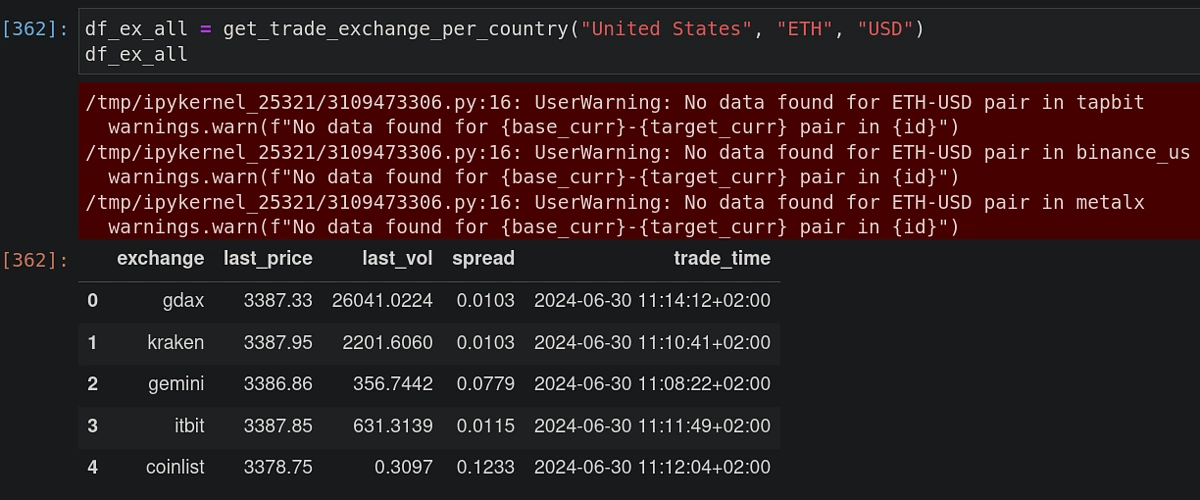
Aggregating and Displaying Data
Aggregation Over Time
When running an arbitrage bot, aggregating data over trading intervals is crucial. Group the data by exchange and calculate relevant statistics: agg_data = tickers_df.groupby(‘exchange’).agg({ ‘last’: ‘mean’, ‘bid’: ‘mean’, ‘ask’: ‘mean’, ‘spread’: ‘mean’, ‘volume’: ‘sum’, ‘converted_volume’: ‘sum’ }).reset_index() Highlight exchanges with maximum and minimum prices: def highlight_extremes(data, col): if col == ‘last’: high = data[‘last’].max() low = data[‘last’].min() data[‘highlight’] = data[‘last’].apply(lambda x: ‘background-color: green’ if x == low else (‘background-color: red’ if x == high else ”)) return data highlighted_df = highlight_extremes(agg_data, ‘last’) highlighted_df.style.applymap(lambda x: x, subset=[‘highlight’])Running the Crypto Exchange Arbitrage Bot
Continuous Monitoring
For continuous monitoring, use a loop to update the data at specified intervals: while True: ticker_data = []for exchange_id in selected_exchanges: tickers = get_ticker_data(exchange_id) eth_usd_ticker = next((t for t in tickers if t['base'] == 'ETH' and t['target'] == 'USD'), {}) if eth_usd_ticker: eth_usd_ticker['exchange'] = exchange_id ticker_data.append(eth_usd_ticker) else: print(f"Ticker not found on ") tickers_df = pd.DataFrame(ticker_data) tickers_df['spread'] = (tickers_df['ask'] - tickers_df['bid']) / tickers_df['ask'] * 100 agg_data = tickers_df.groupby('exchange').agg({ 'last': 'mean', 'bid': 'mean', 'ask': 'mean', 'spread': 'mean', 'volume': 'sum', 'converted_volume': 'sum' }).reset_index() clear_output(wait=True) display(agg_data.sort_values(by='spread')) time.sleep(60) # delay in seconds
Stopping the Bot
To halt the bot’s execution, simply interrupt the kernel within Jupyter by navigating to the “Kernel” tab and selecting “Interrupt Kernel”.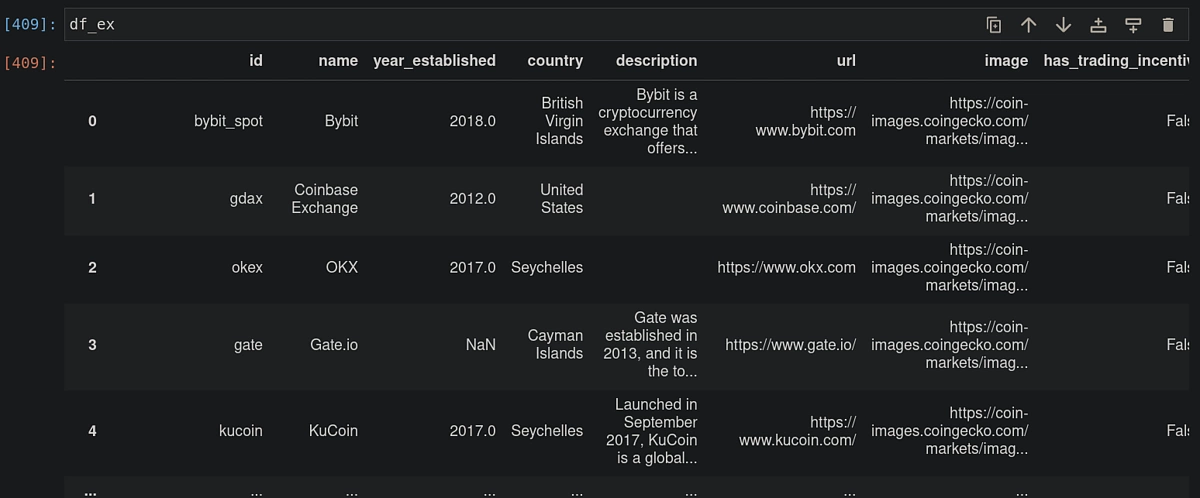
Conclusion
Leveraging Python and CoinGecko’s API within a Jupyter notebook, you can efficiently monitor multiple crypto exchanges to identify arbitrage opportunities. While this guide does not cover trade execution, it forms a solid foundation upon which more comprehensive trading strategies can be built. If you found this guide useful, be sure to explore our extensive collection of other API guides and resources. Happy trading!Investment Intelligence: Your Guide to Mastering Stocks, Cryptocurrencies, and Market Trends with Stockcoin.net
In the dynamic world of finance, timely and accurate information is essential to success. Stockcoin.net exists at the cutting edge of investment intelligence, providing the most up-to-date insights on stocks, cryptocurrencies, bitcoin, and investment trends available online. Available 24/7 and free for life, Stockcoin.net is here to empower investors to make well-informed decisions in real time. Whether you're an experienced trader or just starting out, our platform is designed to provide the tools, data, and resources necessary for you to succeed in today’s market.
Why Choose Stockcoin.net for Your Investment Journey?
Stockcoin.net isn’t just a platform; it’s a financial ally that provides essential resources for investors in a rapidly changing market. By delivering real-time data faster than other sites, we aim to ensure every user has a competitive edge. From stock insights and crypto updates to broader market trends, we offer unmatched resources for those who prioritize informed investment choices. Subscribe to Stockcoin.net for direct email updates, bringing the latest market movements directly to your inbox. By staying connected, you never miss critical information that can shape your investment outcomes.
The Value of Real-Time Information in the Financial Market
The markets move at a relentless pace, and delays can mean missed opportunities. Real-time information is crucial, particularly in fast-paced sectors like cryptocurrency, where values fluctuate by the second. Stockcoin.net prides itself on delivering accurate, real-time updates across multiple sectors, so whether you’re investing in traditional stocks or venturing into digital assets like bitcoin, you’re always equipped with the latest market data. Our site covers:
- Up-to-the-minute stock prices for leading companies and indices.
- Crypto prices and trends for coins such as Bitcoin, Ethereum, and emerging altcoins.
- Investment strategies that help you interpret market shifts.
- Exclusive news alerts delivered directly to your inbox.
By consolidating market data and trends from around the world, Stockcoin.net enables you to make better, faster investment decisions.
Navigating Stock Investment: Strategies and Insights
The world of stocks offers vast potential for growth, but it also requires careful strategy and awareness. Stockcoin.net provides insight into:
- Market Analysis – Regular reports and commentary on sector trends, including technology, healthcare, finance, and more.
- Company-Specific News – Profiles on publicly traded companies, their financial performance, and projected growth.
- Stock Market Indices – Tracking major indices such as the Dow Jones, NASDAQ, and S&P 500 to give you a comprehensive market overview.
Through in-depth analysis and expert perspectives, Stockcoin.net supports you in developing a portfolio that aligns with your financial goals, balancing growth potential with risk management.
Unlocking Cryptocurrency Opportunities
Cryptocurrency has evolved from a niche market to a global financial powerhouse. The rapid development of digital currencies, coupled with the decentralized nature of blockchain technology, presents unique opportunities and risks. Stockcoin.net covers everything you need to know about crypto, including:
- Bitcoin and Ethereum: Comprehensive coverage of the two biggest cryptocurrencies, tracking value changes and providing predictions based on expert analysis.
- Altcoin Developments: Insights on emerging cryptocurrencies, such as Solana, Cardano, and Polkadot, giving you an edge in discovering undervalued assets.
- Blockchain Technology: How blockchain innovations impact investment potential and security.
- Regulatory Updates: Cryptocurrency regulations change quickly, with significant implications for market stability. Our platform provides timely updates to ensure you’re compliant and well-informed.
Whether you’re a seasoned investor looking to diversify or a newcomer eager to explore digital currencies, Stockcoin.net offers the resources you need to make confident decisions in the volatile crypto market.
The Importance of Diversification in Today’s Market
Investing wisely means diversifying your portfolio to manage risk and optimize returns. Stockcoin.net assists users in crafting a diversified investment strategy, providing insights into various asset classes:
- Stocks – Traditional assets, with options for both high-growth and stable income through dividends.
- Cryptocurrencies – High-risk, high-reward assets with potential for exponential gains.
- Bonds – Fixed-income securities that provide stability and lower volatility.
- Commodities – Tangible assets like gold, silver, and oil, which can act as a hedge against inflation.
By offering extensive information on each category, Stockcoin.net empowers you to design a portfolio that suits your risk tolerance, financial goals, and investment timeline.
Staying Ahead with Market Trends and Financial News
In the investment world, being well-informed is essential to avoid costly mistakes and seize potential opportunities. Stockcoin.net offers 24/7 financial news coverage and insightful trend analysis to ensure that you’re always ahead of the curve. Our news section is continuously updated with the latest on:
- Global Market Movements – Real-time updates on stock exchanges around the world, including the NYSE, London Stock Exchange, and Tokyo Stock Exchange.
- Economic Indicators – Regularly updated reports on interest rates, inflation rates, and economic policies that impact global and regional markets.
- Investor Sentiment – Analysis of how consumer confidence and market sentiment can affect asset prices, helping you understand market psychology.
- Sector-Specific Developments – Deep dives into how industries like technology, finance, and energy are evolving.
This data empowers you to spot trends early, make proactive investment choices, and mitigate risks effectively.
Learning from Successful Investors
Stockcoin.net showcases success stories and case studies from users who have achieved notable financial success through our platform. These real-world experiences provide practical insights, highlighting strategies, challenges, and solutions employed by seasoned investors. Learning from their journeys can inspire you to navigate your investment path more effectively.
Avoiding Common Investment Pitfalls
Investing involves risks, and even experienced investors can face setbacks. Stockcoin.net’s mission is to educate users on avoiding common mistakes, such as:
- Overtrading: Buying and selling frequently without clear strategies can lead to unnecessary losses.
- Failing to Diversify: Relying heavily on a single asset or sector can increase vulnerability to market shifts.
- Ignoring Market Sentiment: Market psychology plays a significant role in asset prices; understanding this is crucial.
- Emotional Decisions: Making choices based on fear or greed often results in suboptimal outcomes.
With Stockcoin.net’s expert resources and guidance, you’ll be equipped to build a sustainable investment strategy that withstands market volatility.
The Power of Subscribing to Stockcoin.net
By subscribing to Stockcoin.net, you gain access to exclusive updates and insights delivered directly to your email. These updates ensure you’re among the first to receive critical news, analysis, and investment tips, helping you stay proactive and ready for action. Our subscribers benefit from:
- Instant News Alerts – Stay on top of essential market updates, including sudden price movements and regulatory changes.
- Personalized Investment Tips – Gain tailored suggestions based on your preferences and investment goals.
- Exclusive Resources – Access in-depth reports and analyses not available on our main site.
With a simple email subscription, you can ensure that you’re never out of the loop on the latest financial developments.
Building Financial Confidence with Stockcoin.net
At Stockcoin.net, we’re committed to providing high-quality, accurate, and timely information that enables you to make informed decisions. From understanding the basics of investing to exploring advanced strategies, Stockcoin.net is here as a reliable resource. Our comprehensive coverage of stocks, cryptocurrencies, and market trends positions you to take control of your financial future.
Begin your journey today with Stockcoin.net – the platform that delivers investment intelligence faster and more accurately than any competitor. Subscribe, explore, and make your mark in the world of investing.
- The Art of Being Duped: A Wildean Reflection
- The Allure of Filmy Zdarma Bez Prihlaseni
- Personal Financial Planning Through the Lens of Presidential Policies
- Understanding the Concept of ROE: Maximizing Life's Returns on Effort
- The Art of Being Earnestly Amused by Filmy Online Zdarma
- The Aesthetic Alchemy of a Makeup Kit
- The Dashing Dilemma of Dopt and Desire
- The Art of Wandering: Enigmatic Turistické Destinace
- The Duplicitous Art of Charm
- Ethereum Dominates Stablecoin Liquidity with a Staggering Share
- The Charm of Filmy Zdarma
- The Cadre of Exquisite Eccentricities
- Understanding the Concept of Return on Effort (ROE) for Personal Growth
- The Art of Vanity: A Cosmetic Canvas
- The Allure of Cosmetics: A Wilde Exploration of Beauty and Artifice
- The Dandy's Ode to Dop
- Swiss Church Controversy Surrounds AI Jesus Taking Confessions
- The Perspicacity of Persuasion
- The Dandy's Delight: Havaianas and the Artistry of Leisure
- The Curious Charms of the Department in Disarray
- The Aesthetics of Beauty and the Pursuit of Pleasure
- Understanding the Concept of Return on Effort in Personal Growth
- The Enigmatic Portal of Vanity
- Le Chat Could Kill ChatGPT-4o with This Unique Selling Proposition
- The Art of Personal Vanity: A Wildean Perspective
- The Art of the Signature: A Study in Elegant Flourish
- The Importance of a Plagiarism Checker in the Quest for Originality
- The Signature of Fortune in a Crypto Casino
- The Art of Deception: A Crypto Casino's Plagiarism Checker
- Overview of 2025 401(k) Contribution Limits
- Best 50+ Real money slot apps for android
- Best 50+ Unveiling the Secrets of Coin Casinos
- Best 50+ Maximize Your Winnings at Gamble Max Casino
- Exploring the Impact of Sunshine Sweeps Casino Best 50+
- Sky sweeps casino real money no deposit bonus Top 50+
- Top 50+ Real Bitcoin Casinos in the USA
- Top 50+ Anonymous Casino Bonuses for Players
- Top 10+ Bitcoin Games to Play Now
- Best 50+ The Rise of BTC Gambling Platforms
- Real usa bitcoin casino free spins Best 50+
- Best 50+ Slot games that pay real money to cash app
- Benefits of Playing at No Verification Casinos Top 50+
- Best 50+ Top Casino Apps for Mobile Gaming
- Best 50+ Free Online Fruit Machines
- Best 50+ Slot games that pay real money to cash app
- Benefits of Playing at No Verification Casinos Top 50+
Stockcoin.net: Your Trusted Source for Financial Clarity and Insight
In today’s digital age, finding reliable, accurate, and timely financial information is a challenge. Stockcoin.net shines as a dependable resource amid a sea of misinformation, dedicated to delivering the most accurate, real-time financial insights across stocks, cryptocurrencies, and investment trends. With a focus on clarity and accessibility, Stockcoin.net is tailored to both seasoned investors and beginners seeking to make sense of complex financial landscapes.
The Origins of Stockcoin.net: Born from the Need for Speed and Accuracy
Founded by a team of financial experts and tech innovators, Stockcoin.net was developed to bridge a crucial gap in the world of financial news and data: timeliness. Traditional news sources often lag, leaving investors scrambling for information and potentially making decisions based on outdated data. Stockcoin.net’s mission is to remedy this by offering instantaneous updates and in-depth analyses, ensuring users are equipped to act on the latest information.
Meeting the Needs of Today’s Investors
Stockcoin.net understands that access to up-to-date and accurate information is essential for investors. Our platform provides a comprehensive suite of tools that are designed to help users stay informed, including:
- Real-Time Stock Quotes: Live updates on major stock indices, blue-chip stocks, and emerging market opportunities.
- Comprehensive Cryptocurrency Coverage: Detailed insights into Bitcoin, Ethereum, and trending altcoins, ensuring you’re always on top of the latest in the crypto sphere.
- In-Depth Market Analyses: Market trends, economic indicators, and forecasts curated by financial experts and updated around the clock.
Introduction to Stockcoin.net: Your Investment Ally
Navigating financial data can be challenging, particularly for those new to investing. Stockcoin.net’s intuitive interface is designed with user-friendliness in mind, ensuring easy access to essential information. From stock quotes to in-depth crypto analyses, every feature is accessible with just a few clicks, streamlining the investment experience.
Accessibility and Affordability at the Core
Stockcoin.net is free for life, a choice rooted in our commitment to making financial literacy available to everyone. We believe that access to accurate financial information shouldn’t be a privilege but a standard. With a simple email subscription, users can receive personalized alerts and updates directly in their inbox, ensuring they’re always informed and ready to act on new developments.
Educational Resources to Empower Every Investor
At Stockcoin.net, we believe that knowledge is power in the investment world. Financial literacy is crucial for success, so we’ve curated a comprehensive library of educational content that supports both novice and advanced investors. Our educational offerings include:
- Articles and Tutorials: From introductory guides on stock trading to advanced crypto investment strategies, our resources are designed for every skill level.
- Webinars and Workshops: Live and recorded sessions hosted by financial experts that dive deep into trending topics and investment techniques.
- Investment Glossary and FAQs: A full glossary of terms and answers to common questions for those looking to build their foundational knowledge.
Our mission is to ensure every investor, regardless of their experience, can navigate the complexities of financial markets with confidence.
The Critical Role of Real-Time Alerts in Modern Investing
In the fast-paced world of finance, every second counts. Delayed reactions to market changes can lead to missed opportunities or, worse, financial losses. Stockcoin.net’s real-time alerts provide users with immediate notifications on significant market movements, empowering them to make timely investment decisions. These alerts cover a wide range of data points, including:
- Price Changes in Stocks and Cryptocurrencies: Real-time notifications on shifts in stock prices and cryptocurrency values.
- Breaking Financial News: Instant updates on global economic events, company earnings, and regulatory changes.
- Investment Recommendations: Tailored insights and strategy suggestions based on current market conditions.
This feature is particularly valuable in the volatile cryptocurrency market, where prices can swing dramatically in minutes. With Stockcoin.net, you’re always prepared to take advantage of these movements.
Stockcoin.net’s Key Features: A Closer Look at What Sets Us Apart
Stockcoin.net is a comprehensive financial resource, combining cutting-edge technology with expert insights to create a platform that stands out in the crowded financial information space. Here’s what makes Stockcoin.net unique:
1. Real-Time Market Data and Analysis
Whether you’re tracking stocks, cryptocurrencies, or economic indicators, Stockcoin.net provides real-time updates and expert analysis. Our team curates insights from the global financial landscape, ensuring users are always one step ahead.
2. Personalized Investment Tools
We understand that each investor has unique goals, so our tools allow users to customize their experience. From tracking specific stocks and cryptocurrencies to receiving tailored news based on your portfolio, Stockcoin.net provides a personalized touch that makes investing simpler and more effective.
3. Community of Like-Minded Investors
With our educational resources and community features, Stockcoin.net fosters a network where investors can share insights, experiences, and strategies. Users can learn from one another and grow their knowledge alongside fellow investors.
4. Comprehensive Market Coverage
Stockcoin.net covers the full spectrum of investment opportunities, including traditional stocks, ETFs, mutual funds, and alternative assets like commodities. Our wide-ranging analyses provide users with a holistic view of market opportunities, enabling them to make diversified and balanced investment decisions.
Subscribing to Stockcoin.net: Staying Ahead with Email Alerts
The financial world changes rapidly, and staying updated is crucial. By subscribing to Stockcoin.net, you’ll receive personalized alerts and daily insights directly to your inbox, ensuring you’re always informed. Benefits of subscribing include:
- Instant Access to Exclusive News and Analyses: Be among the first to receive critical updates that may impact your portfolio.
- Customized Alerts for Key Market Movements: Stay on top of price changes and market trends specific to your areas of interest.
- Weekly Market Summaries: Comprehensive reviews of the past week’s performance across stocks, cryptocurrencies, and key indices, giving you a complete market picture.
A Trusted Partner in Your Financial Journey
Investing can be complex, but Stockcoin.net is here to guide you every step of the way. With a platform designed to simplify financial information and empower users through knowledge, we aim to be more than a source of data; we are your partner in financial success. By delivering timely, accurate, and actionable insights, Stockcoin.net positions you to make informed, confident decisions as you navigate the investment world.
As you explore the resources on Stockcoin.net, embrace the opportunities that come with timely information and expert insights. Your journey with Stockcoin.net is one of growth, education, and success in the financial markets. Start leveraging our tools and resources today, and watch as your investment strategies evolve with the power of knowledge and clarity.
What is the stockcoin.net website?
Our Stockcoin.net website delivers the latest information such as stocks, cryptocurrencies, bitcoin, and investment faster and more accurate than anyone else. Stockcoin.net website is free for life and is available for 24 hours. If you subscribe to our website by entering the email below, you will be able to receive the latest investment information by emailing us at the earliest.
In today's fast-paced financial landscape, information is a trader's most valuable asset. Welcome to "Investment Intelligence," your essential guide to navigating the intricate world of stocks, cryptocurrencies, and market trends. At the heart of this exploration is Stockcoin.net, a revolutionary platform dedicated to delivering the latest investment information faster and more accurately than any competitor. Whether you’re a seasoned investor or a curious beginner, this book aims to empower you with the knowledge and tools necessary to make informed decisions.
Stockcoin.net is more than just a website; it's a comprehensive resource designed for individuals who seek to stay ahead in the ever-evolving market environment. With the stock market constantly fluctuating and new cryptocurrencies emerging daily, having access to real-time, accurate information is paramount. Our platform is accessible 24/7, providing you with the insights needed to seize opportunities as they arise, all free for life.
Through this book, we will delve into various aspects of investing. Chapter by chapter, we will explore fundamental concepts, analyze market dynamics, and examine investment strategies that align with modern trends. From understanding the basics of stocks to the intricacies of cryptocurrency trading, each chapter is crafted to build your financial literacy and confidence.
You will discover how to interpret market data, assess risks, and develop a diversified portfolio that can withstand the volatility of financial markets. Additionally, we will discuss the importance of staying updated with timely information, which can be a game-changer in your investment journey. By subscribing to Stockcoin.net, you will receive personalized updates directly to your inbox, ensuring that you never miss crucial news that could impact your investments.
Furthermore, we will share success stories from users who have harnessed the power of our platform to achieve their financial goals. These case studies will provide real-world insights and inspire you to take action. You'll learn from their experiences, mistakes, and triumphs as they navigated the investment landscape.
In this book, we also address common pitfalls that many investors face and offer guidance on how to avoid them. The world of investing can be intimidating, but with the right knowledge and resources, it can also be incredibly rewarding. Our goal is to equip you with the tools you need to make sound investment choices and achieve your financial objectives.
As we embark on this journey together, remember that the key to successful investing lies not just in knowledge, but also in the continuous pursuit of information. With Stockcoin.net as your ally, you will have a wealth of resources at your fingertips, empowering you to take control of your financial future.
Let’s dive into the first chapter, where we will introduce you to Stockcoin.net and the myriad ways it can enhance your investment experience.
Introduction to Stockcoin.net: Your Investment Ally
In an era where information is abundant yet often misleading, having a reliable source of financial news and data is crucial. Stockcoin.net stands out as a beacon of clarity in this complex landscape. Our mission is to provide users with the most accurate and up-to-date information regarding stocks, cryptocurrencies, and investment trends. This chapter will explore the inception of Stockcoin.net, its features, and the value it brings to investors worldwide.
Founded by a team of financial experts and technology enthusiasts, Stockcoin.net was born out of a necessity for speed and accuracy in financial reporting. Traditional financial news outlets often lag behind real-time developments, leaving investors at a disadvantage. Recognizing this gap, we set out to create a platform that delivers timely insights, enabling users to make decisions based on the latest data.
One of the core features of Stockcoin.net is its user-friendly interface. We understand that navigating financial information can be overwhelming, especially for beginners. Thus, our website is designed to be intuitive, allowing users to easily access the information they need. From real-time stock quotes to in-depth analyses of cryptocurrencies, everything is just a click away.
In addition to providing accurate information, we also prioritize accessibility. Stockcoin.net is free for life, ensuring that everyone, regardless of their financial situation, can benefit from our resources. We believe that financial literacy should not be a privilege; it should be available to all. By subscribing with your email, you can receive personalized updates and insights directly in your inbox, allowing you to stay ahead of market trends.
Another standout feature of Stockcoin.net is our commitment to education. We recognize that informed investors are successful investors. Therefore, we offer a wealth of educational content, including articles, tutorials, and webinars, designed to enhance your understanding of financial markets. Whether you're looking to grasp the basics of stock trading or dive deep into advanced cryptocurrency strategies, our resources cater to every level of expertise.
Moreover, the importance of real-time information cannot be overstated. In financial markets, timing can make all the difference. With Stockcoin.net, you’ll receive alerts about significant market movements, enabling you to react swiftly to changes that may impact your investments. This feature is particularly vital in the cryptocurrency space, where volatility is the norm.
As we progress through this chapter, we will delve deeper into the specific features of Stockcoin.net that set it apart from other platforms. From our comprehensive market analyses to user-friendly tools that help you track your investments, every aspect is designed with your success in mind.
Ultimately, Stockcoin.net is more than just a tool; it's a partner in your investment journey. As you explore the chapters ahead, we invite you to leverage the resources available through our platform. Embrace the possibilities that come with timely information and enhanced financial literacy, and watch as your investment strategies evolve.
Subscribe to Blog via Email
Table of Contents
ToggleDiscover more from Stockcoin.net
Subscribe to get the latest posts sent to your email.